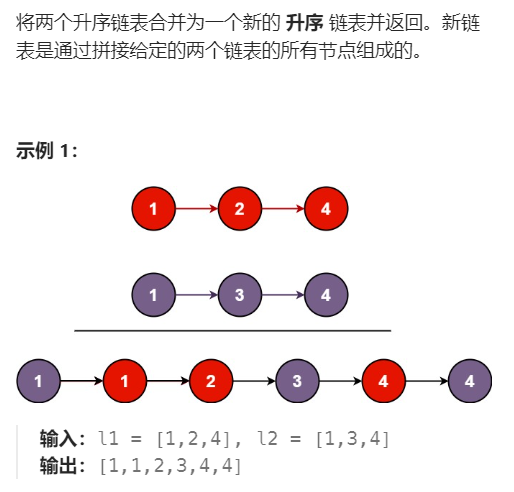
递归
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} list1
* @param {ListNode} list2
* @return {ListNode}
*/
var mergeTwoLists = function (l1, l2) {
if (!l1) return l2
if (!l2) return l1
if (l1.val < l2.val) {
l1.next = mergeTwoLists(l1.next, l2);
return l1;
} else {
l2.next = mergeTwoLists(l1, l2.next);
return l2;
}
};
暴力解法/迭代
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} list1
* @param {ListNode} list2
* @return {ListNode}
*/
var mergeTwoLists = function (l1, l2) {
const prehead = new ListNode(-1);
let prev = prehead;
while (l1 != null && l2 != null) {
if (l1.val <= l2.val) {
prev.next = l1;
l1 = l1.next;
} else {
prev.next = l2;
l2 = l2.next;
}
prev = prev.next;
}
// 合并后 l1 和 l2 最多只有一个还未被合并完,我们直接将链表末尾指向未合并完的链表即可
prev.next = l1 === null ? l2 : l1;
return prehead.next;
};
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} list1
* @param {ListNode} list2
* @return {ListNode}
*/
var mergeTwoLists = function (list1, list2) {
if (!list1) return list2
if (!list2) return list1
let temp1 = list2
while (list2) {
if (!list1) break
if (!list2?.next) {
if (list1.val < list2.val) {
const temp = list2.next
list2.next = list1
list1 = list1.next
list2.next.next = temp
} else {
list2.next = list1
break
}
} else if (list1.val <= list2.next.val) {
const temp = list2.next
list2.next = list1
list1 = list1.next
list2.next.next = temp
list2 = list2.next
} else {
list2 = list2.next
}
}
const temp2 = temp1
if (temp1 && temp1.next && temp1.val > temp1.next.val) {
while (temp1.next && temp1.val > temp1.next.val) {
const a = temp1.val
temp1.val = temp1.next.val
temp1.next.val = a
temp1 = temp1.next
}
}
return temp2
};
评论区